How to create WordPress like button without login/plugin
Posted on by ChandanIn this article we will learn to create WordPress like button with counts based on IP address of the visitor (so, no login is required to like your posts/pages). You can use this on both posts and pages without plugin. Also Its very good practice to create it without using a plugin so that you can modify it very easily.
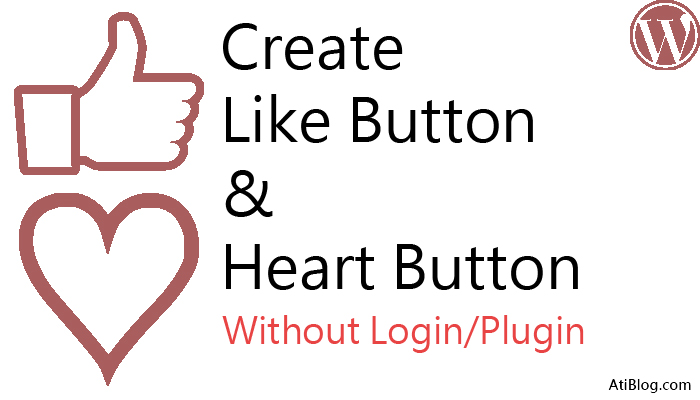
Here we will discuss every step in order to build this code for like button. Also you can replace this to heart button by just changing the icon used.
What are the things we will use in our post like system?
- General PHP
- WordPress Database handling ($wpdb)
- jQuery
- WordPress Admin Ajax (for logged or not logged in users)
- A normal 2016 theme , where we will add our code into.
What is the general Algorithm for this?
- First of all, we create a html code for the like button and add it where we want our btn. In our case we can add this to template-parts/content-single.php in our 2016 theme. Also we will add CSS for the styles.
- Furthermore, in the theme’s js folder, we will add a javascript file , where all the ajax and js works will be written. So please add a file Twenty Sixteen/js/post-like.js . We will enqueue this js file from the functions.php.
- Finally, in our functions.php , we will do the following things:
-> Create a table which will store the data like postid and userip for each click on the like/ heart button. You can create a table from phpmyadmin too, but I did this from functions.php.
-> Enqueue the script file used to process the AJAX. Also the localization of script.
->A function which will be used to process the AJAX calls on every click.
The code for HTML part what we add in our posts / page for the like button to appear:
We use fontawesome to create the button.
<style>
a.pp_like i {
font-size: 18px;
color: #007acc;
}
a.pp_like {
text-decoration: none;
box-shadow: none;
}
a.pp_like.liked i {
color: red;
}
.lds-facebook {
display: inline-block;
position: relative;
width: 64px;
height: 38px;
display:none;
margin-top: -20px;
}
.lds-facebook div {
display: inline-block;
position: absolute;
left: 6px;
width: 13px;
background: #007acc;
animation: lds-facebook 1.2s cubic-bezier(0, 0.5, 0.5, 1) infinite;
}
.lds-facebook div:nth-child(1) {
left: 0px;
animation-delay: -0.24s;
}
.lds-facebook div:nth-child(2) {
left: 22px;
animation-delay: -0.12s;
}
.lds-facebook div:nth-child(3) {
left: 45px;
animation-delay: 0;
}
@keyframes lds-facebook {
0% {
top: 6px;
height: 51px;
}
50%, 100% {
top: 19px;
height: 26px;
}
}
</style>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.5.0/css/all.css" integrity="sha384-B4dIYHKNBt8Bc12p+WXckhzcICo0wtJAoU8YZTY5qE0Id1GSseTk6S+L3BlXeVIU" crossorigin="anonymous">
<?php
global $wpdb;
$l=0;
$postid=get_the_id();
$clientip=get_client_ip();
$row1 = $wpdb->get_results( "SELECT id FROM $wpdb->post_like_table WHERE postid = '$postid' AND clientip = '$clientip'");
if(!empty($row1)){
$l=1;
}
$totalrow1 = $wpdb->get_results( "SELECT id FROM $wpdb->post_like_table WHERE postid = '$postid'");
$total_like1 = $wpdb->num_rows;
?>
<div class="post_like">
<a class="pp_like <?php if($l==1) {echo "liked"; } ?>" href="#" data-id="<?php echo get_the_id(); ?>"><i class="fas fa-thumbs-up"></i> <span><?php echo $total_like1; ?> like</span></a>
<div class="lds-facebook"><div></div><div></div><div></div></div>
</div>
The post-like.js code (handling the click on like button):
jQuery(document).ready(function($) {
jQuery('.pp_like').click(function(e){
e.preventDefault();
jQuery('.pp_like').hide();
jQuery('.lds-facebook').show();
var postid=jQuery(this).data('id');
//alert(postid);
var data = {
action: 'my_action',
security : MyAjax.security,
postid: postid
};
jQuery.post(MyAjax.ajaxurl, data, function(res) {
var result=jQuery.parseJSON( res );
console.log(result);
//alert(res);
var likes="";
likes=result.likecount + " like";
jQuery('.pp_like span').text(likes);
if(result.like ==1){
jQuery('.pp_like').addClass('liked');
}
if(result.dislike ==1){
jQuery('.pp_like').removeClass('liked');
}
jQuery('.pp_like').show();
jQuery('.lds-facebook').hide();
});
});
});
The code to add in the functions.php file:
function post_like_table_create() {
global $wpdb;
$table_name = $wpdb->prefix. "post_like_table";
global $charset_collate;
$charset_collate = $wpdb->get_charset_collate();
global $db_version;
if( $wpdb->get_var("SHOW TABLES LIKE '" . $table_name . "'") != $table_name)
{ $create_sql = "CREATE TABLE " . $table_name . " (
id INT(11) NOT NULL auto_increment,
postid INT(11) NOT NULL ,
clientip VARCHAR(40) NOT NULL ,
PRIMARY KEY (id))$charset_collate;";
require_once(ABSPATH . "wp-admin/includes/upgrade.php");
dbDelta( $create_sql );
}
//register the new table with the wpdb object
if (!isset($wpdb->post_like_table))
{
$wpdb->post_like_table = $table_name;
//add the shortcut so you can use $wpdb->stats
$wpdb->tables[] = str_replace($wpdb->prefix, '', $table_name);
}
}
add_action( 'init', 'post_like_table_create');
// Add the JS
function theme_name_scripts() {
wp_enqueue_script( 'script-name', get_template_directory_uri() . '/js/post-like.js', array('jquery'), '1.0.0', true );
wp_localize_script( 'script-name', 'MyAjax', array(
// URL to wp-admin/admin-ajax.php to process the request
'ajaxurl' => admin_url( 'admin-ajax.php' ),
// generate a nonce with a unique ID "myajax-post-comment-nonce"
// so that you can check it later when an AJAX request is sent
'security' => wp_create_nonce( 'my-special-string' )
));
}
add_action( 'wp_enqueue_scripts', 'theme_name_scripts' );
// The function that handles the AJAX request
function get_client_ip() {
$ip=$_SERVER['REMOTE_ADDR'];
return $ip;
}
function my_action_callback() {
check_ajax_referer( 'my-special-string', 'security' );
$postid = intval( $_POST['postid'] );
$clientip=get_client_ip();
$like=0;
$dislike=0;
$like_count=0;
//check if post id and ip present
global $wpdb;
$row = $wpdb->get_results( "SELECT id FROM $wpdb->post_like_table WHERE postid = '$postid' AND clientip = '$clientip'");
if(empty($row)){
//insert row
$wpdb->insert( $wpdb->post_like_table, array( 'postid' => $postid, 'clientip' => $clientip ), array( '%d', '%s' ) );
//echo $wpdb->insert_id;
$like=1;
}
if(!empty($row)){
//delete row
$wpdb->delete( $wpdb->post_like_table, array( 'postid' => $postid, 'clientip'=> $clientip ), array( '%d','%s' ) );
$dislike=1;
}
//calculate like count from db.
$totalrow = $wpdb->get_results( "SELECT id FROM $wpdb->post_like_table WHERE postid = '$postid'");
$total_like=$wpdb->num_rows;
$data=array( 'postid'=>$postid,'likecount'=>$total_like,'clientip'=>$clientip,'like'=>$like,'dislike'=>$dislike);
echo json_encode($data);
//echo $clientip;
die(); // this is required to return a proper result
}
add_action( 'wp_ajax_my_action', 'my_action_callback' );
add_action( 'wp_ajax_nopriv_my_action', 'my_action_callback' );
[…] We have done something similar here: How to create WordPress like button without login/plugin […]