WordPress include tags, categories and taxonomies in search results
Posted on by ChandanMany times we need to search by associated tags, category or custom taxonomy names . But WordPress doesn’t provide these by default. Therefore, we need to make our own results page which include the default search including tags, categories and custom taxonomy names. In this article, we learn a technique or a guideline to tackle these terms and show the matching posts if they have these.
For example, If you have ‘mytag’ in some of your posts and you want to perform_search on ‘mytag’ the result should show that post.
First of all if your theme doesn’t have search.php, then just add this file in your activated theme. This file is used to show the results.
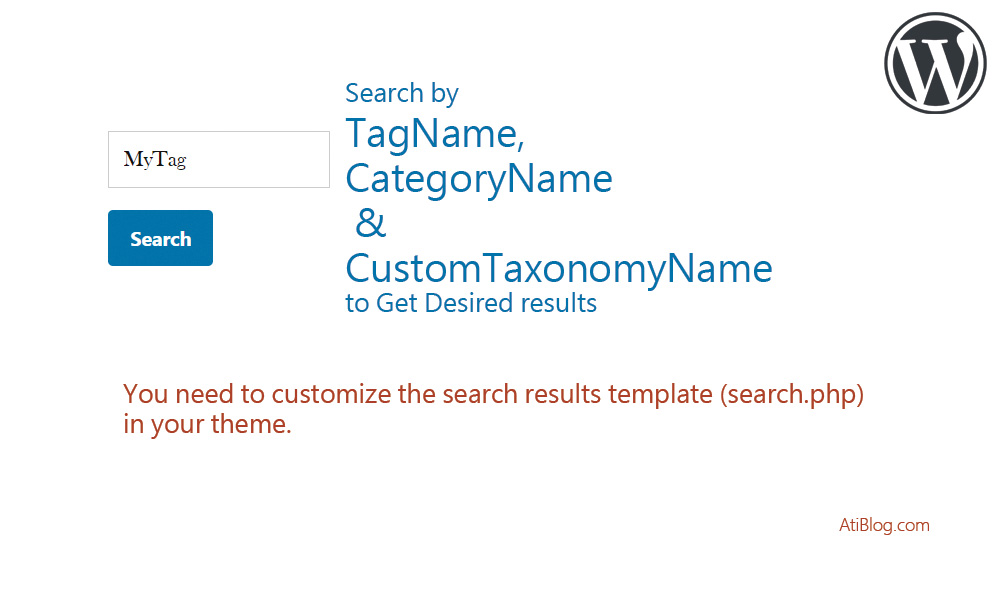
In our Example, we will use the wordpress default theme twenty nineteen and modify search.php to get the desired results. This theme doesn’t include tags and categories for matching the keyphrase . We change everything on search.php file.
Basic Idea behind our code example:
- Remove the old query in the search.php file.
- get all the category, tag and custom taxonomy in array format. And loop every array and find matching name in these arrays. Then store the ids of the matched name in a separate array.
- Using wp_query, query all the posts (by tax query )with these matched ids. Then store the resulting post_ids in an array.
- Now perform a default search_query and store all the ids in an array.
- Now we have got two arrays of ids which is the result.
- Combine these two and remove duplicates.
- Now we will perform a last query and show all the posts (by post__in parameter of wp_query) .
<?php
/**
* The template for displaying search results pages
*
* @link https://developer.wordpress.org/themes/basics/template-hierarchy/#search-result
*
* @package WordPress
* @subpackage Twenty_Nineteen
* @since 1.0.0
*/
get_header();
?>
<section id="primary" class="content-area">
<main id="main" class="site-main">
<header class="page-header">
<h1 class="page-title">
<?php _e( 'Search results for:', 'twentynineteen' ); ?>
</h1>
<div class="page-description"><?php echo get_search_query(); ?></div>
</header><!-- .page-header -->
<?php
$search=get_search_query();
$all_categories = get_terms( array('taxonomy' => 'category','hide_empty' => true) );
$all_tags = get_terms( array('taxonomy' => 'post_tag','hide_empty' => true) );
//if you have any custom taxonomy
$all_custom_taxonomy = get_terms( array('taxonomy' => 'your-taxonomy-slug','hide_empty' => true) );
$mcat=array();
$mtag=array();
$mcustom_taxonomy=array();
foreach($all_categories as $all){
$par=$all->name;
if (strpos($par, $search) !== false) {
array_push($mcat,$all->term_id);
}
}
foreach($all_tags as $all){
$par=$all->name;
if (strpos($par, $search) !== false) {
array_push($mtag,$all->term_id);
}
}
foreach($all_custom_taxonomy as $all){
$par=$all->name;
if (strpos($par, $search) !== false) {
array_push($mcustom_taxonomy,$all->term_id);
}
}
$matched_posts=array();
$args1= array(
'post_status' => 'publish',
'posts_per_page' => -1,
'tax_query' => array(
'relation' => 'OR',
array(
'taxonomy' => 'category',
'field' => 'term_id',
'terms' => $mcat
),
array(
'taxonomy' => 'post_tag',
'field' => 'term_id',
'terms' => $mtag
),
array(
'taxonomy' => 'custom_taxonomy',
'field' => 'term_id',
'terms' => $mcustom_taxonomy
)
)
);
$the_query = new WP_Query( $args1 );
if ( $the_query->have_posts() ) {
while ( $the_query->have_posts() ) {
$the_query->the_post();
array_push($matched_posts,get_the_id());
//echo '<li>' . get_the_id() . '</li>';
}
wp_reset_postdata();
} else {
}
?>
<?php
// now we will do the normal wordpress search
$query2 = new WP_Query( array( 's' => $search,'posts_per_page' => -1 ) );
if ( $query2->have_posts() ) {
while ( $query2->have_posts() ) {
$query2->the_post();
array_push($matched_posts,get_the_id());
}
wp_reset_postdata();
} else {
}
$matched_posts= array_unique($matched_posts);
$matched_posts=array_values(array_filter($matched_posts));
//print_r($matched_posts);
?>
<?php
$paged = ( get_query_var('paged') ) ? get_query_var('paged') : 1;
$query3 = new WP_Query( array( 'post_type'=>'any','post__in' => $matched_posts ,'paged' => $paged) );
if ( $query3->have_posts() ) {
while ( $query3->have_posts() ) {
$query3->the_post();
get_template_part( 'template-parts/content/content', 'excerpt' );
}
twentynineteen_the_posts_navigation();
wp_reset_postdata();
} else {
}
?>
</main><!-- #main -->
</section><!-- #primary -->
<?php
get_footer();
WP Resources :
Similar article based on searching attachment images with ajax
There are some plugins available to do this but doing this custom is very helpful to modify thing. Therefore we are doing this without using a plugin.
[…] WordPress include tags, categories and taxonomies in search results […]